Planetarium
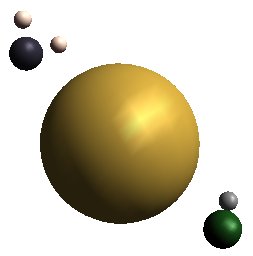
The code that renders the planets are like this.
public void drawPlanets(GL gl) { GLU glu = new GLU(); // spheres GLUquadric glpQ=glu.gluNewQuadric(); /** the sun in origo */ /** Material of the sun*/ someMaterials.SetMaterialGoldenSun(gl); /** radius 4 */ glu.gluSphere(glpQ,4.0f,20,20); gl.glPushMatrix(); /** a planet */ /** This planets material */ someMaterials.setMaterialGreenPlanet(gl); /** in XY-plane */ gl.glRotatef(m_pV,1.0f,0.0f,0.0f); /** distance 7 */ gl.glTranslatef(0.0f,7.0f,0.0f); /** radius 1 */ glu.gluSphere(glpQ,1.0f,20,20); /** with its only moon */ /** This moons material */ someMaterials.setMaterialSilverMoon(gl); /** in YZ-pane (of planet) */ gl.glRotatef(m_mV,1.0f,0.0f,0.0f); /** distance 1.5 */ gl.glTranslatef(0.0f,1.5f,0.0f); /** radius 0.5 */ glu.gluSphere(glpQ,0.5f,20,20); gl.glPopMatrix(); gl.glPushMatrix(); /** another planet */ /** This planets material */ someMaterials.setMaterialObsidianPlanet(gl); /** in XZ-plane */ gl.glRotatef(m_pV2,0.0f,1.0f,0.0f); /** distance 10 */ gl.glTranslatef(10.0f,0.0f,0.0f); /** radius 1 */ glu.gluSphere(glpQ,1.0f,20,20); gl.glPushMatrix(); /** with its first moon */ /** These moons material */ someMaterials.setMaterialWhiteMoon(gl); /** in XZ-pane (of planet) */ gl.glRotatef(m_mV2,0.0f,1.0f,0.0f); /** distance 3 */ gl.glTranslatef(3.0f,0.0f,0.0f); /** radius 0.5 */ glu.gluSphere(glpQ,0.5f,20,20); gl.glPopMatrix(); gl.glPushMatrix(); /** and second moon */ /** in XY-pane (of planet) */ gl.glRotatef(m_mV2,0.0f,0.0f,1.0f); /** distance 2 */ gl.glTranslatef(2.0f,0.0f,0.0f); /** radius 0.5 */ glu.gluSphere(glpQ,0.5f,20,20); gl.glPopMatrix(); gl.glPopMatrix(); glu.gluDeleteQuadric(glpQ); // increment model movement m_Vz += 0.5f; if (m_Vz > 360.0f) m_Vz = 0.5f; m_Vx += 0.1f; if (m_Vx > 360.0f) m_Vx = 0.1f; m_pV += 0.1f; if (m_pV > 360.0f) m_pV = 0.1f; m_mV += 0.9f; if (m_mV > 360.0f) m_mV = 0.9f; m_pV2 += 0.2f; if (m_pV2 > 360.0f) m_pV2 = 0.2f; m_mV2 += 0.9f; if (m_mV2 > 360.0f) m_mV2 = 0.0f; }
Note that you can stop the movements with right mousebutton.